题目描述
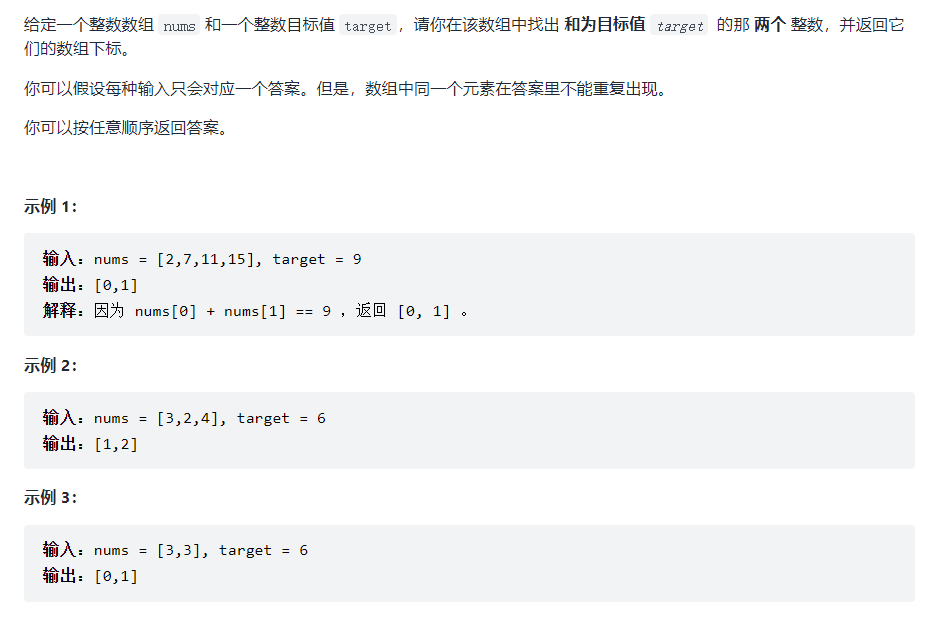
解法一(暴力穷举法)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public int[] twoSum(int[] nums, int target) { int[] result=new int[2]; for (int i = 0; i <nums.length ; i++) { for (int j = i+1; j <nums.length; j++) { if(nums[i]+nums[j]==target){ result[0]=i; result[1]=j; return result; } } } return result; } }
|
- 时间复杂度:O(N^2),其中 NN 是数组中的元素数量。最坏情况下数组中任意两个数都要被匹配一次。
- 空间复杂度:O(1)。
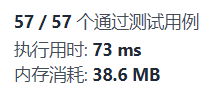
解法二(通过HashMap键值对实现)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| class Solution { public int[] twoSum(int[] nums, int target) { int[] result=new int[2]; Map<Integer,Integer> map=new HashMap<>(); for (int i = 0; i < nums.length; i++) { int another=target-nums[i]; Integer isNum=map.get(another); if(isNum!=null){ result[0]=isNum; result[1]=i; break; } else map.put(nums[i],i); } return result; } }
|
思路
使用哈希表,将元素的值与下标存进哈希表,循环遍历数组时 通过**target-num[i]**找到所需数的key然后通过哈希表查找,没有则将该数和下标存进哈希表。此时只需要一层for循环
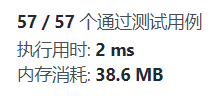
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/two-sum