题目描述
给你一个二叉树的根节点 root
,按 任意顺序 ,返回所有从根节点到叶子节点的路径。
叶子节点 是指没有子节点的节点。
示例 1:
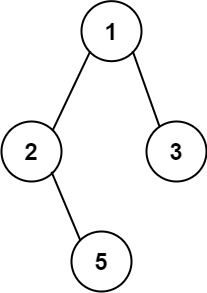
1 2
| 输入:root = [1,2,3,null,5] 输出:["1->2->5","1->3"]
|
示例 2:
提示:
- 树中节点的数目在范围
[1, 100]
内
-100 <= Node.val <= 100
解法
- 深度优先搜索
- 如果当前节点不是叶子节点,则在当前的路径末尾添加该节点,并继续递归遍历该节点的每一个孩子节点。
- 如果当前节点是叶子节点,则在当前路径末尾添加该节点后我们就得到了一条从根节点到叶子节点的路径,将该路径加入到答案即可。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
|
class Solution { public List<String> binaryTreePaths(TreeNode root) { List<String> paths=new ArrayList<>(); constructPaths(root, "",paths); return paths; } public void constructPaths(TreeNode root,String path ,List<String> paths){ if(root!=null){ StringBuffer pathSB=new StringBuffer(path); pathSB.append(Integer.toString(root.val)); if(root.left==null&&root.right==null){ paths.add(pathSB.toString()); } else { pathSB.append("->"); constructPaths(root.left, pathSB.toString(), paths); constructPaths(root.right, pathSB.toString(), paths); } } } }
|
来源:力扣(LeetCode)
链接:257. 二叉树的所有路径 - 力扣(LeetCode)