题目描述
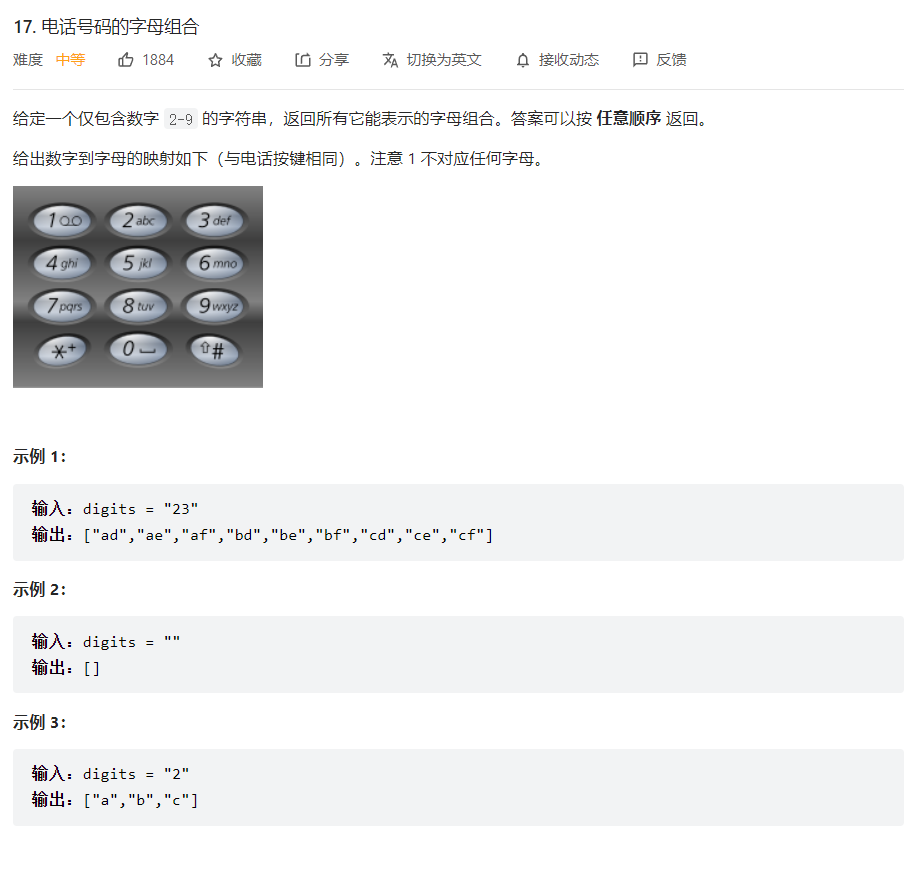
解法
- 回溯,深度优先搜索
- 题目要求给出所有的组合结果,使用回溯,进行结果的遍历
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| class Solution { public List<String> letterCombinations(String digits) { List<String> combinations=new ArrayList<>(); if (digits.length()==0){ return combinations; } Map<Character,String> phoneMap=new HashMap<>(); phoneMap.put('2', "abc"); phoneMap.put('3', "def"); phoneMap.put('4', "ghi"); phoneMap.put('5', "jkl"); phoneMap.put('6', "mno"); phoneMap.put('7', "pqrs"); phoneMap.put('8', "tuv"); phoneMap.put('9', "wxyz"); backtrack(combinations, phoneMap, digits, 0, new StringBuffer()); return combinations; }
public void backtrack(List<String> combinations,Map<Character,String> phoneMap,String digits,int index,StringBuffer combinBuffer){ if(index==digits.length()){ combinations.add(combinBuffer.toString()); } else { char digit=digits.charAt(index); String letters=phoneMap.get(digit); int lettersCount=letters.length(); for (int i = 0; i < lettersCount; i++) { combinBuffer.append(letters.charAt(i)); backtrack(combinations, phoneMap, digits, index+1, combinBuffer); combinBuffer.deleteCharAt(index); } } } }
|
来源:力扣(LeetCode)
链接:17. 电话号码的字母组合 - 力扣(LeetCode)